
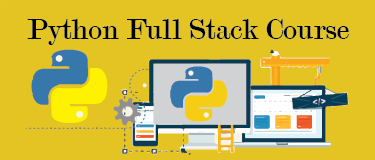
This way it doesn't matter if input events come with little time between them, they'll all be stored and available for processing. We can enqueue all input events as they come in. A tester noticed that if buttons are pressed too quickly the game only processes the first one and special moves won't work! In your game, every time a button is pressed, an input event is fired. Now imagine that you're a developer working on a new fighting game. These button combinations can be stored in a queue. Players in those games can perform special moves by pressing a combination of buttons. Think of games like Street Fighter or Super Smash Brothers. Queues have widespread uses in programming as well. # The title is better on the left with bold fontĭocument_actions.push( 'action: format text_id: 1 style: bold') # As with most writers, the user is unhappy with the first draft and undoes the center alignment # The first enters the title of the documentĭocument_actions.push( 'action: enter text_id: 1 text: This is my favorite document')ĭocument_actions.push( 'action: format text_id: 1 alignment: center') We can quickly simulate the feature like this: When the user wants to undo an action they'll pop it from the stack.
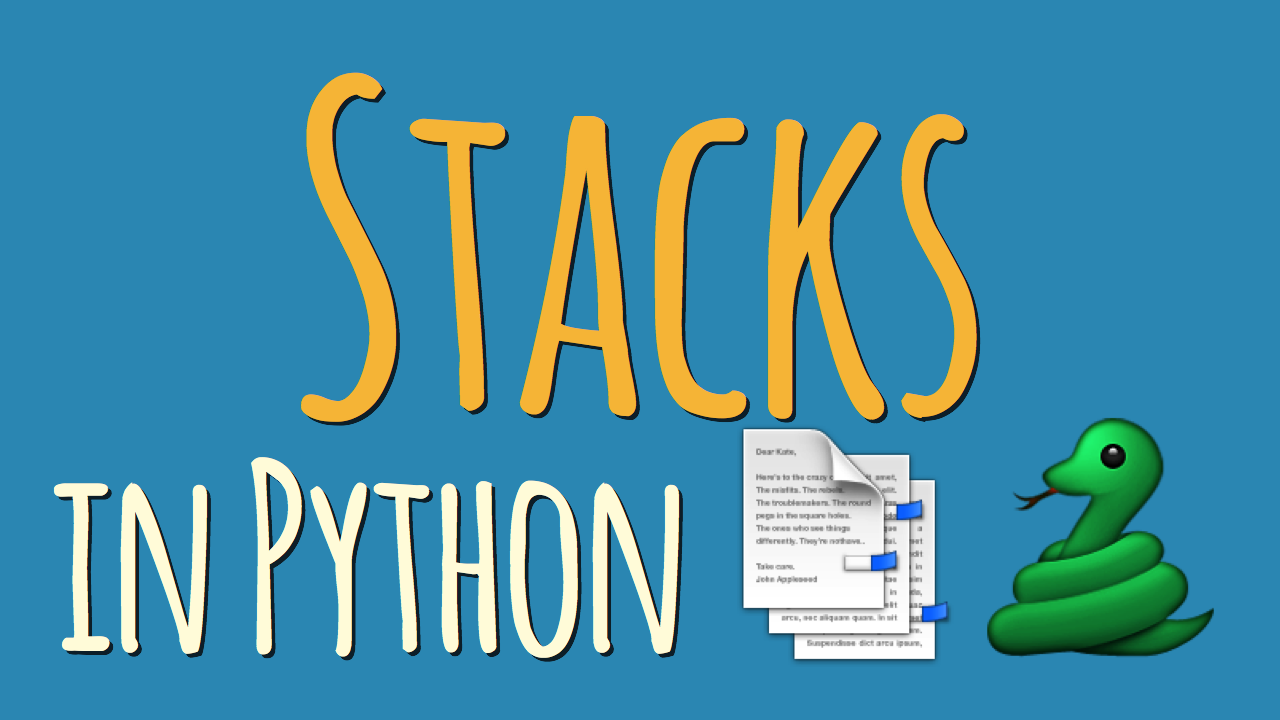
We can record every action the user takes by pushing it to the stack. You're tasked with creating an undo feature - allowing users to backtrack their actions till the beginning of the session.Ī stack is an ideal fit for this scenario. Imagine you're a developer working on a brand new word processor.
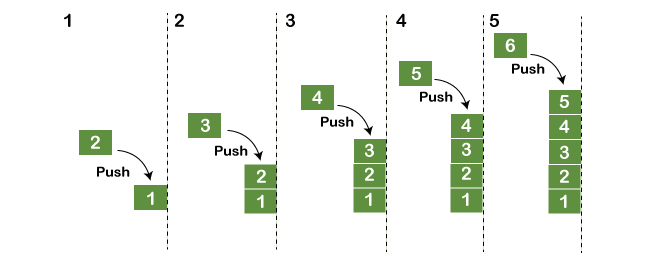
The programmers using our Stack and Queue are now encouraged to use the methods provided to manipulate the data instead. # And a queue that only has enqueue and dequeue operations class Queue: def _init_( self):ĭef dequeue( self): if len(self.queue) < 1: To do so, let's create a new file called stack_queue.py and define two classes: # A simple class stack that only allows pop and push operations class Stack: def _init_( self): We can create classes that only expose the necessary methods for each data structure. There are times when we'd like to ensure that only valid operations can be performed on our data.
#CREATE A STACK IN PYTHON CODE#
If your code needs a stack and you provide a List, there's nothing stopping a programmer from calling insert(), remove() or other list methods that will affect the order of your stack! This fundamentally ruins the point of defining a stack, as it no longer functions the way it should. Print(first_item) # 99 print(numbers) # deque()Īdvice: If you'd like to learn more about the deque library and other types of collections Python provides, you can read our "Introduction to Python's Collections Module" article. Print(last_item) # 47 print(numbers) # deque() # You can dequeue like a queue Python has a deque (pronounced 'deck') library that provides a sequence with efficient methods to work as a stack or a queue.ĭeque is short for Double Ended Queue - a generalized queue that can get the first or last element that's stored: from collections import deque # 'mango' and 'orange' remain print(fruits) # Īgain, here we use the append and pop operations of the list to simulate the core operations of a queue. # Now let's dequeue our fruits, we should get 'banana' # Let's enqueue some fruits into our list
